LineContext
- Reply API
- Push API
- Quick Replies
- Profile API
- Group/Room Member Profile API
- Group/Room Member IDs API
- Leave API
- Rich Menu API
- Account Link API
Reply API - Official Docs
Responds to events from users, groups, and rooms.
reply(messages)
Responds messages to the receiver using reply token.
Param | Type | Description |
---|---|---|
messages | Object[] | Array of objects which contains the contents of the message to be sent. |
Example:
context.reply([
{
type: 'text',
text: 'Hello!',
},
]);
replyToken
can only be used once, but you can send up to 5 messages using the same token.
const { Line } = require('messaging-api-line');
context.reply([
Line.createText('Hello'),
Line.createImage({
originalContentUrl: 'https://example.com/original.jpg',
previewImageUrl: 'https://example.com/preview.jpg',
}),
Line.createText('End'),
]);
replyText(text)
- Official Docs
Responds text message to the receiver using reply token.
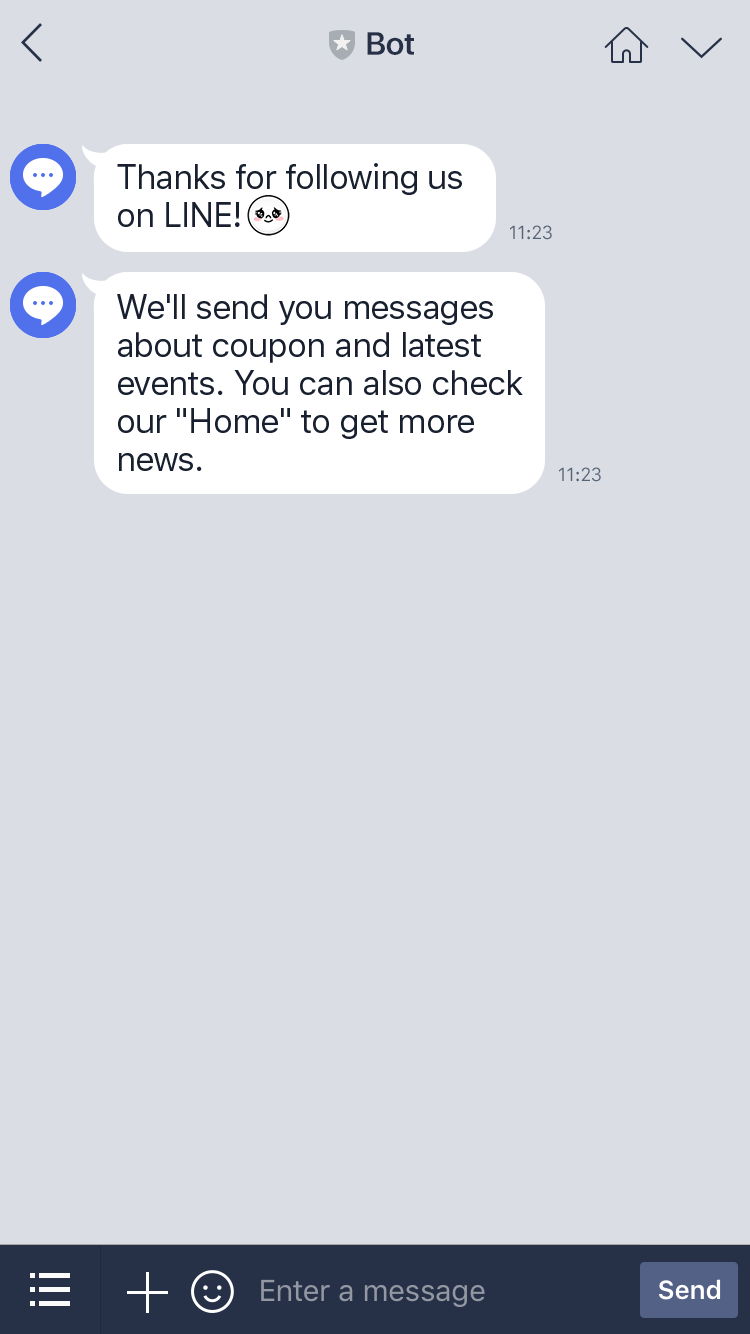
You can include LINE original emoji in text messages using character codes. For a list of LINE emoji that can be sent in LINE chats, see the emoji list.
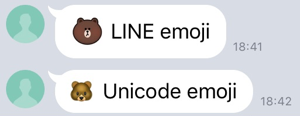
Param | Type | Description |
---|---|---|
text | String | Text of the message to be sent. |
Example:
context.replyText('Hello!');
replyImage(imageUrl, previewImageUrl)
- Official Docs
Responds image message to the receiver using reply token.
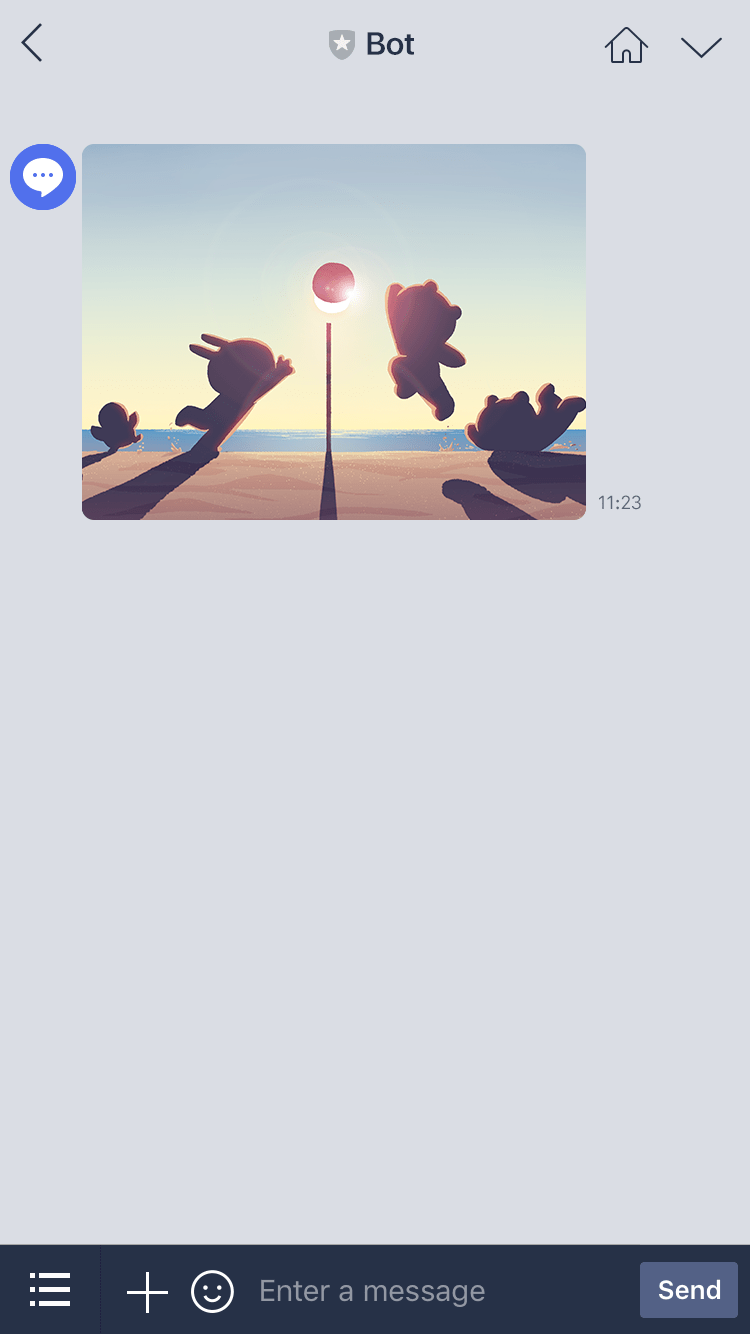
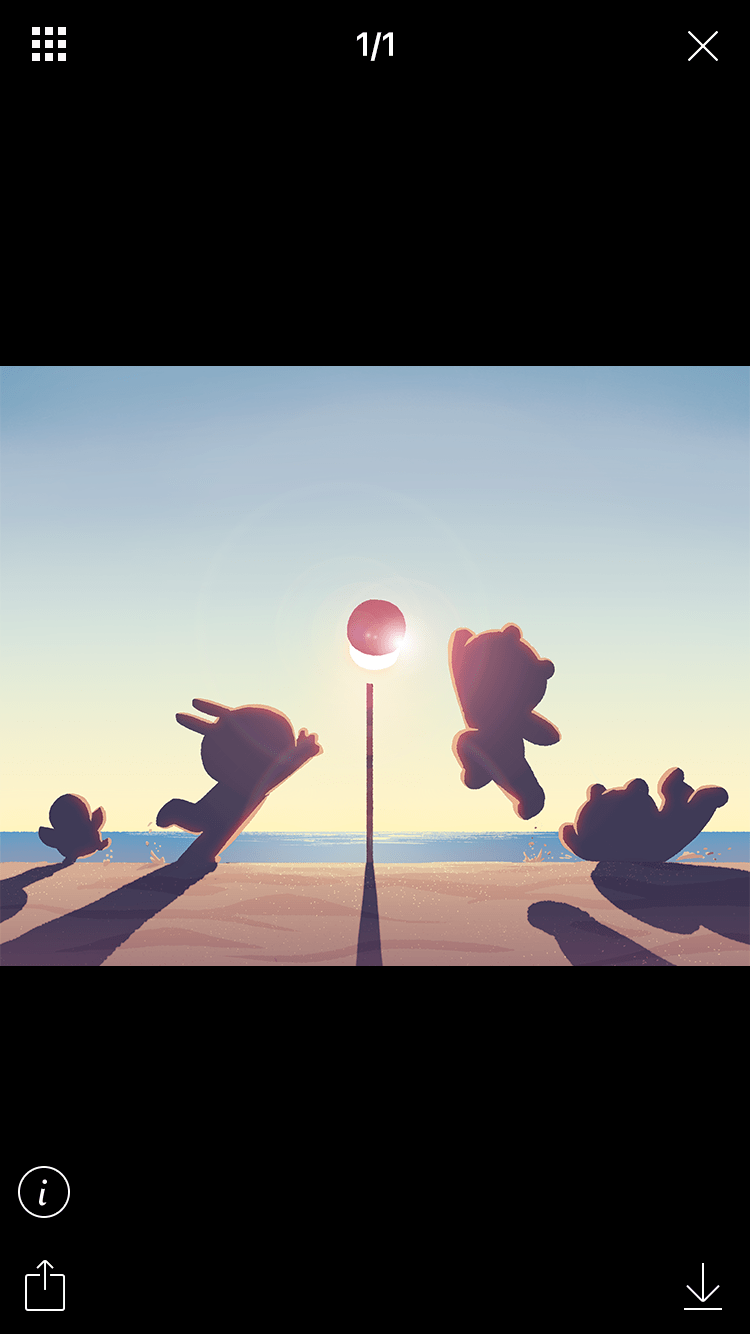
Param | Type | Description |
---|---|---|
imageUrl | String | Image URL. |
previewImageUrl | String | Preview image URL. |
Example:
context.replyImage({
originalContentUrl: 'https://example.com/original.jpg',
previewImageUrl: 'https://example.com/preview.jpg',
});
replyVideo(videoUrl, previewImageUrl)
- Official Docs
Responds video message to the receiver using reply token.
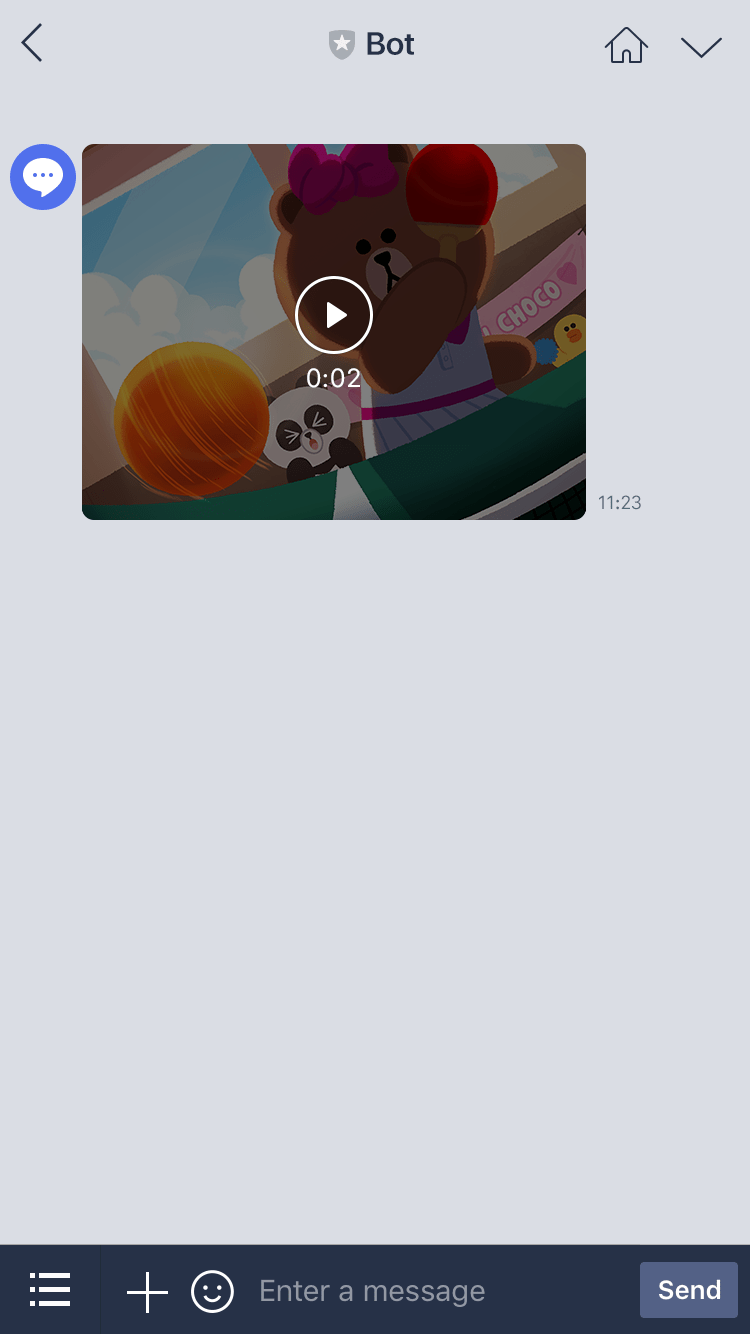
Param | Type | Description |
---|---|---|
videoUrl | String | URL of video file. |
previewImageUrl | String | URL of preview image. |
Example:
context.replyVideo({
originalContentUrl: 'https://example.com/original.mp4',
previewImageUrl: 'https://example.com/preview.jpg',
});
replyAudio(audioUrl, duration)
- Official Docs
Responds audio message to the receiver using reply token.
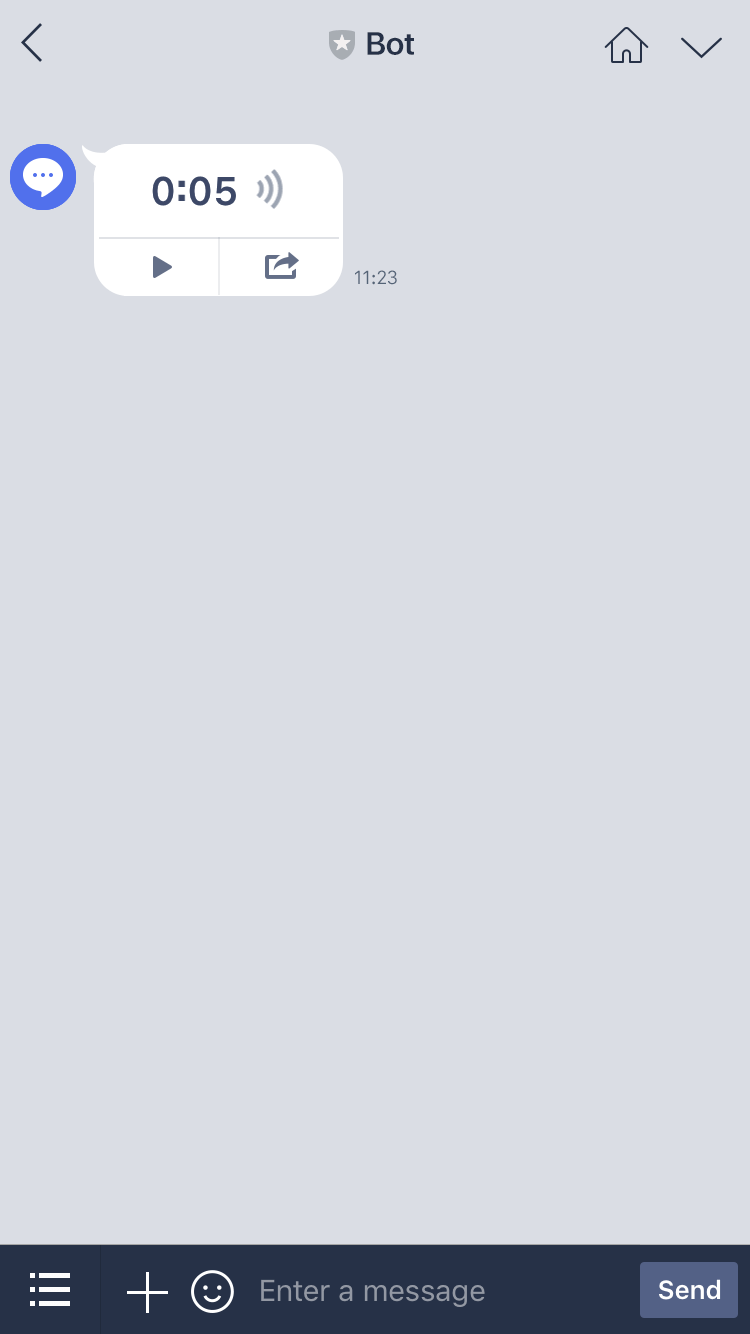
Param | Type | Description |
---|---|---|
audioUrl | String | URL of audio file. |
duration | Number | Length of audio file. |
Example:
context.replyAudio({
originalContentUrl: 'https://example.com/original.m4a',
duration: 240000,
});
replyLocation(location)
- Official Docs
Responds location message to the receiver using reply token.
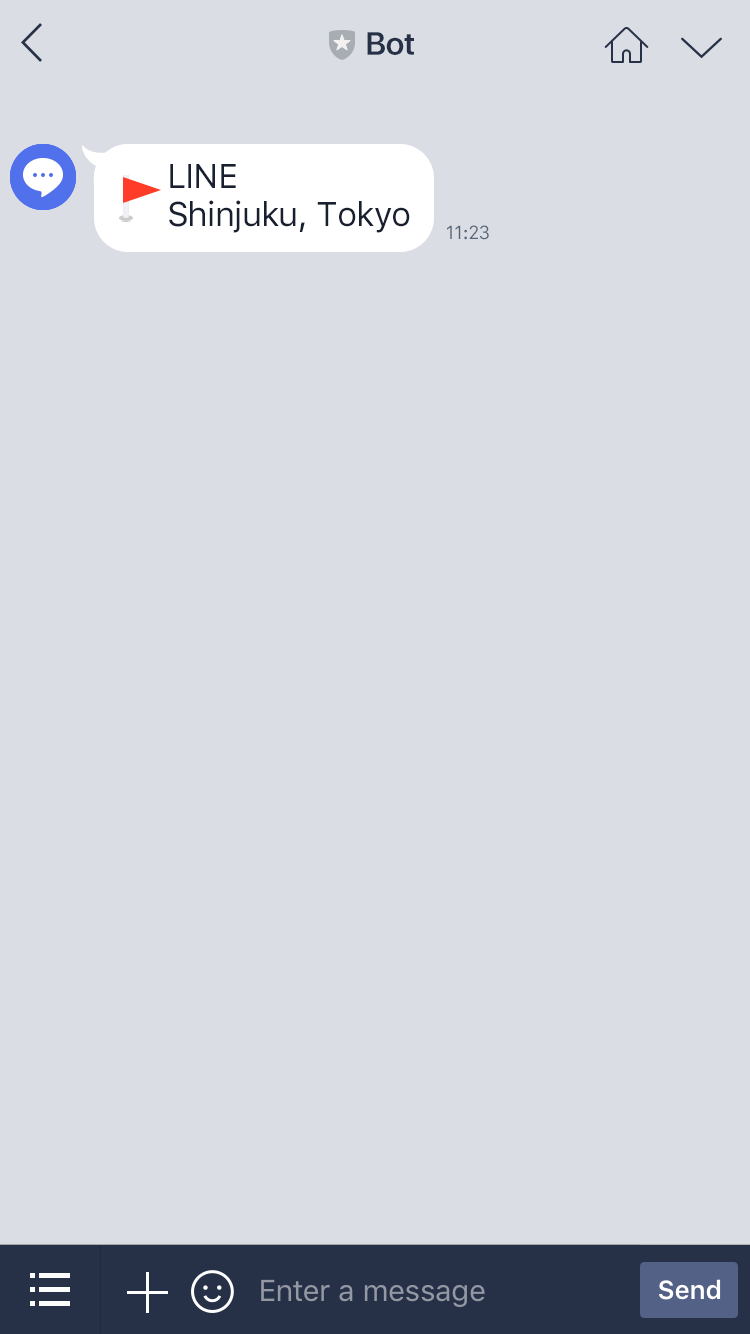
Param | Type | Description |
---|---|---|
location | Object | Object contains location's parameters. |
location.title | String | Title of the location. |
location.address | String | Address of the location. |
location.latitude | Number | Latitude of the location. |
location.longitude | Number | Longitude of the location. |
Example:
context.replyLocation({
title: 'my location',
address: '〒150-0002 東京都渋谷区渋谷2丁目21−1',
latitude: 35.65910807942215,
longitude: 139.70372892916203,
});
replySticker(packageId, stickerId)
- Official Docs
Responds sticker message to the receiver using reply token.
For a list of stickers that can be sent with the Messaging API, see the [sticker list](https://developers.line.me/media/messaging-api/messages/sticker_list.pdf).
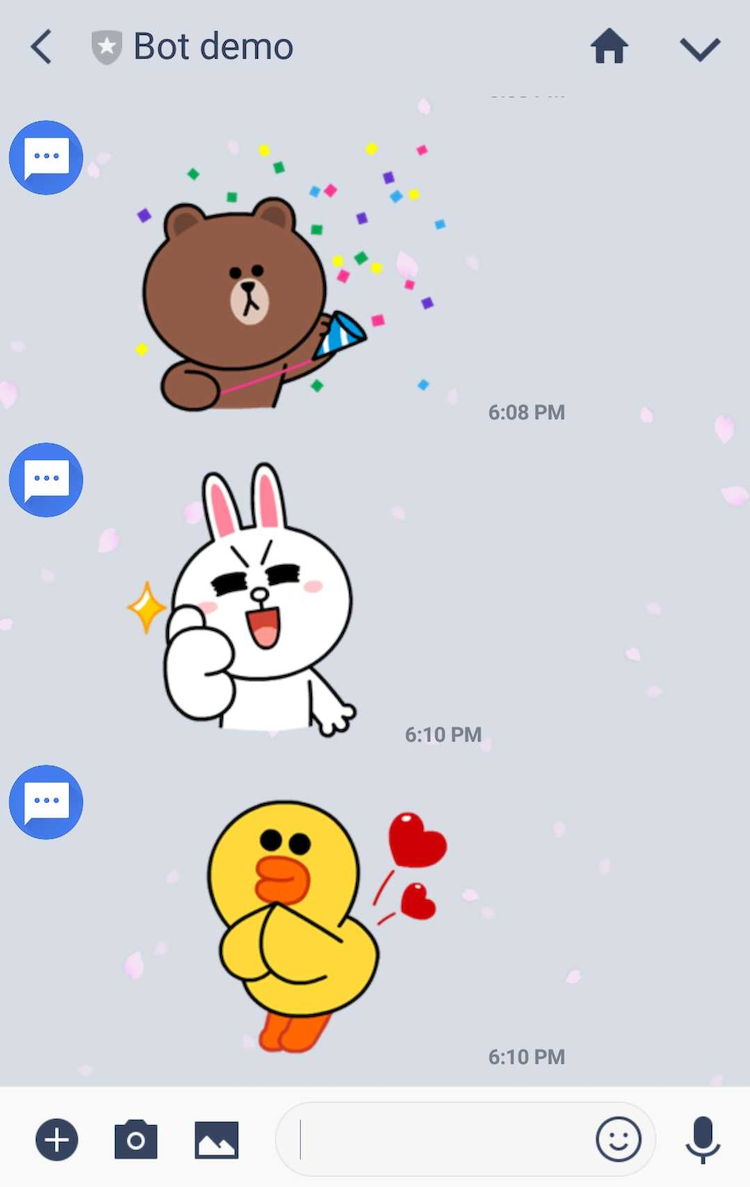
Param | Type | Description |
---|---|---|
packageId | String | Package ID. |
stickerId | String | Sticker ID. |
Example:
context.replySticker({ packageId: '1', stickerId: '1' });
Reply Imagemap Message
replyImagemap(altText, imagemap)
- Official Docs
Responds imagemap message to the receiver using reply token.
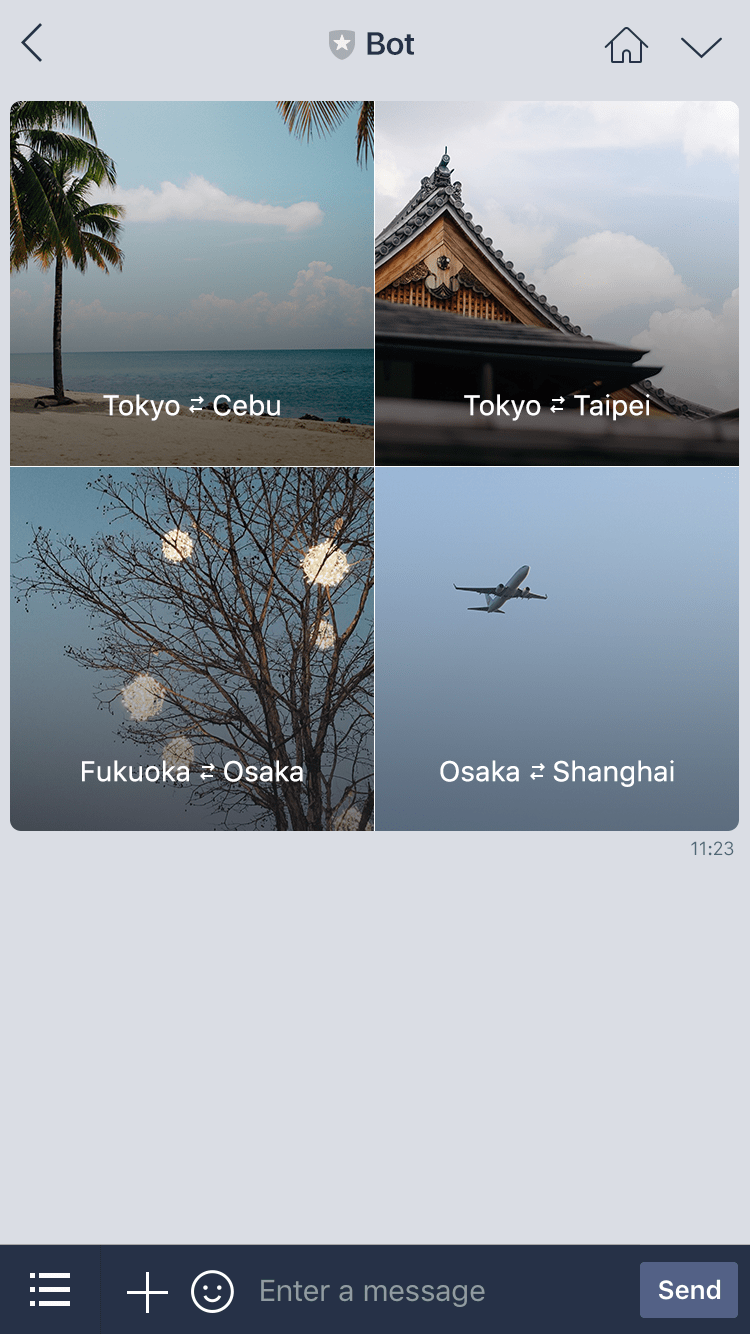
Param | Type | Description |
---|---|---|
altText | String | Alternative text. |
imagemap | Object | Object contains imagemap's parameters. |
imagemap.baseUrl | String | Base URL of image. |
imagemap.baseSize | Object | Base size object. |
imagemap.baseSize.width | Number | Width of base image. |
imagemap.baseSize.height | Number | Height of base image. |
imagemap.actions | Object[] | Action when tapped. |
Example:
context.replyImagemap('this is an imagemap', {
baseUrl: 'https://example.com/bot/images/rm001',
baseSize: {
width: 1040,
height: 1040,
},
actions: [
{
type: 'uri',
linkUri: 'https://example.com/',
area: {
x: 0,
y: 0,
width: 520,
height: 1040,
},
},
{
type: 'message',
text: 'hello',
area: {
x: 520,
y: 0,
width: 520,
height: 1040,
},
},
],
});
Reply Template Messages
replyTemplate(altText, template)
- Official Docs
Responds template message to the receiver using reply token.
Param | Type | Description |
---|---|---|
altText | String | Alternative text. |
template | Object | Object with the contents of the template. |
Example:
context.replyTemplate('this is a template', {
type: 'buttons',
thumbnailImageUrl: 'https://example.com/bot/images/image.jpg',
title: 'Menu',
text: 'Please select',
actions: [
{
type: 'postback',
label: 'Buy',
data: 'action=buy&itemid=123',
},
{
type: 'postback',
label: 'Add to cart',
data: 'action=add&itemid=123',
},
{
type: 'uri',
label: 'View detail',
uri: 'http://example.com/page/123',
},
],
});
replyButtonTemplate(altText, buttonTemplate)
- Official Docs
Alias: replyButtonsTemplate
.
Responds button template message to the receiver using reply token.
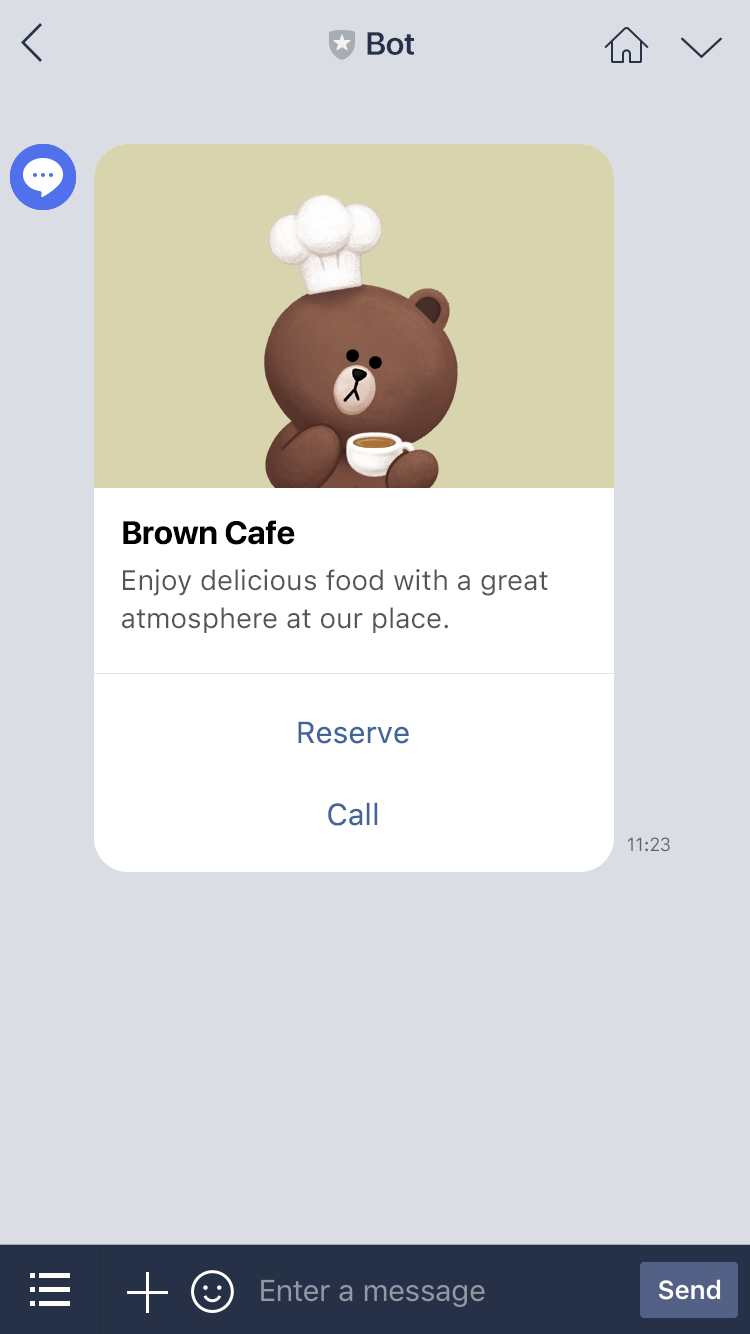
Param | Type | Description |
---|---|---|
altText | String | Alternative text. |
buttonTemplate | Object | Object contains buttonTemplate's parameters. |
buttonTemplate.thumbnailImageUrl | String | Image URL of buttonTemplate. |
buttonTemplate.imageAspectRatio | String | Aspect ratio of the image. Specify one of the following values: rectangle , square |
buttonTemplate.imageSize | String | Size of the image. Specify one of the following values: cover , contain |
buttonTemplate.imageBackgroundColor | String | Background color of image. Specify a RGB color value. The default value is #FFFFFF (white). |
buttonTemplate.title | String | Title of buttonTemplate. |
buttonTemplate.text | String | Message text of buttonTemplate. |
buttonTemplate.defaultAction | Object | Action when image is tapped; set for the entire image, title, and text area. |
buttonTemplate.actions | Object[] | Action when tapped. |
Example:
context.replyButtonTemplate('this is a template', {
thumbnailImageUrl: 'https://example.com/bot/images/image.jpg',
title: 'Menu',
text: 'Please select',
actions: [
{
type: 'postback',
label: 'Buy',
data: 'action=buy&itemid=123',
},
{
type: 'postback',
label: 'Add to cart',
data: 'action=add&itemid=123',
},
{
type: 'uri',
label: 'View detail',
uri: 'http://example.com/page/123',
},
],
});
replyConfirmTemplate(altText, confirmTemplate)
- Official Docs
Responds confirm template message to the receiver using reply token.
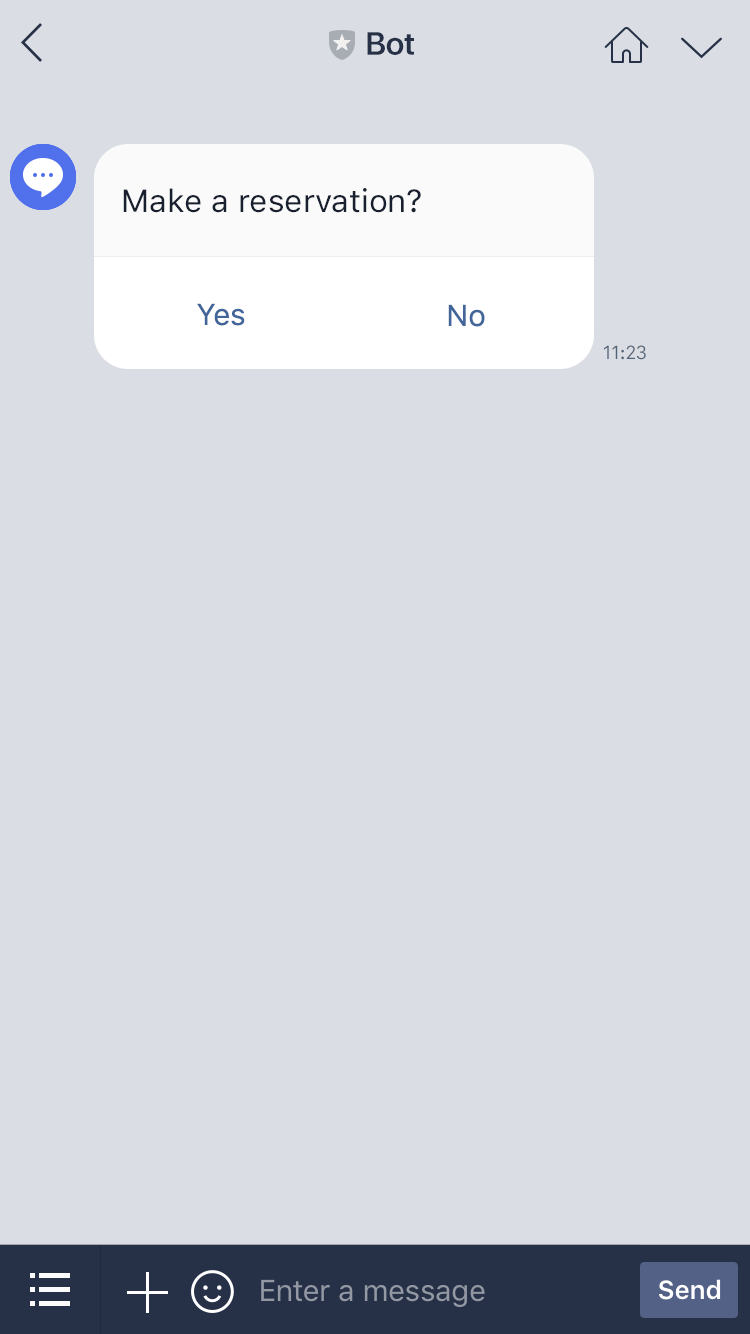
Param | Type | Description |
---|---|---|
altText | String | Alternative text. |
confirmTemplate | Object | Object contains confirmTemplate's parameters. |
confirmTemplate.text | String | Message text of confirmTemplate. |
confirmTemplate.actions | Object[] | Action when tapped. |
Example:
context.replyConfirmTemplate('this is a confirm template', {
text: 'Are you sure?',
actions: [
{
type: 'message',
label: 'Yes',
text: 'yes',
},
{
type: 'message',
label: 'No',
text: 'no',
},
],
});
replyCarouselTemplate(altText, carouselItems, options)
- Official Docs
Responds carousel template message to the receiver using reply token.
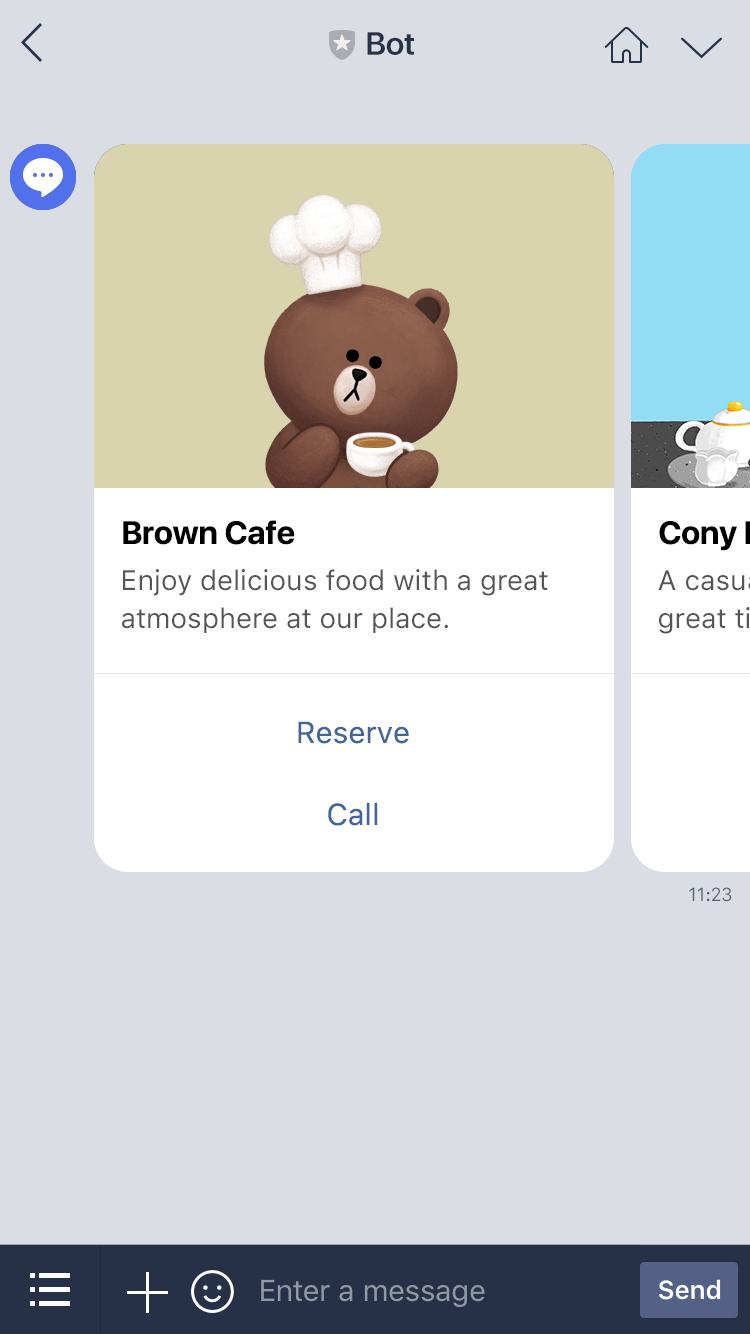
Param | Type | Description |
---|---|---|
altText | String | Alternative text. |
carouselItems | Object[] | Array of columns which contains object for carousel. |
options | Object | Object contains options. |
options.imageAspectRatio | String | Aspect ratio of the image. Specify one of the following values: rectangle , square |
options.imageSize | String | Size of the image. Specify one of the following values: cover , contain |
Example:
context.replyCarouselTemplate('this is a carousel template', [
{
thumbnailImageUrl: 'https://example.com/bot/images/item1.jpg',
title: 'this is menu',
text: 'description',
actions: [
{
type: 'postback',
label: 'Buy',
data: 'action=buy&itemid=111',
},
{
type: 'postback',
label: 'Add to cart',
data: 'action=add&itemid=111',
},
{
type: 'uri',
label: 'View detail',
uri: 'http://example.com/page/111',
},
],
},
{
thumbnailImageUrl: 'https://example.com/bot/images/item2.jpg',
title: 'this is menu',
text: 'description',
actions: [
{
type: 'postback',
label: 'Buy',
data: 'action=buy&itemid=222',
},
{
type: 'postback',
label: 'Add to cart',
data: 'action=add&itemid=222',
},
{
type: 'uri',
label: 'View detail',
uri: 'http://example.com/page/222',
},
],
},
]);
replyImageCarouselTemplate(altText, carouselItems)
- Official Docs
Responds image carousel template message to the receiver using reply token.
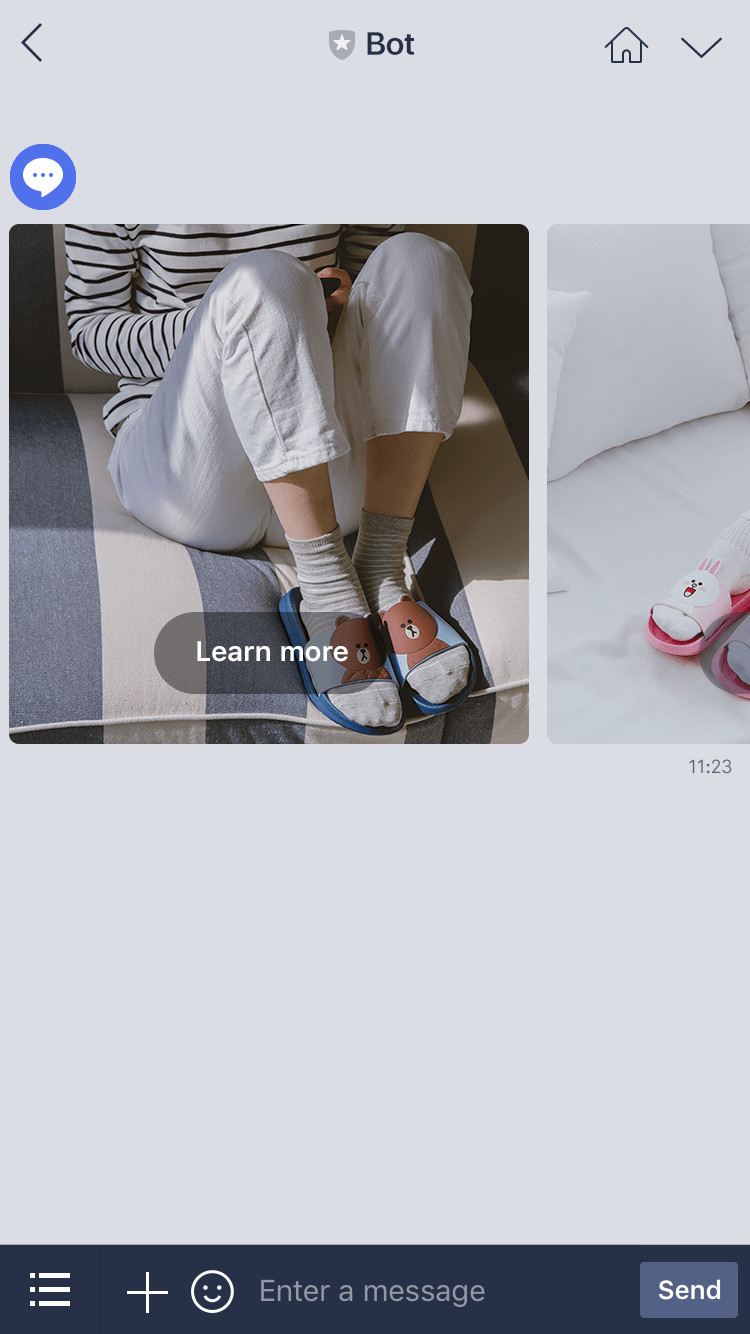
Param | Type | Description |
---|---|---|
altText | String | Alternative text. |
carouselItems | Object[] | Array of columns which contains object for image carousel. |
Example:
context.replyImageCarouselTemplate('this is an image carousel template', [
{
imageUrl: 'https://example.com/bot/images/item1.jpg',
action: {
type: 'postback',
label: 'Buy',
data: 'action=buy&itemid=111',
},
},
{
imageUrl: 'https://example.com/bot/images/item2.jpg',
action: {
type: 'message',
label: 'Yes',
text: 'yes',
},
},
{
imageUrl: 'https://example.com/bot/images/item3.jpg',
action: {
type: 'uri',
label: 'View detail',
uri: 'http://example.com/page/222',
},
},
]);
Reply Flex Messages
replyFlex(altText, contents)
- Official Docs
Responds flex message using specified reply token.
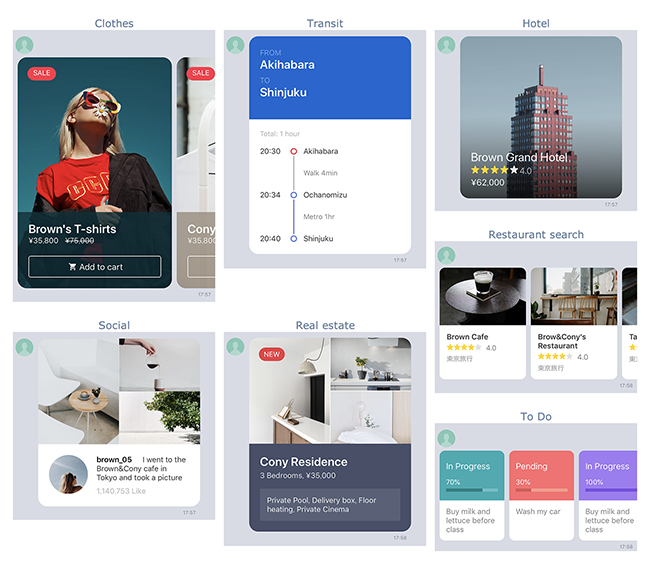
Param | Type | Description |
---|---|---|
altText | String | Alternative text. |
contents | Object | Flex Message container object. |
Example:
context.replyFlex('this is a flex', {
type: 'bubble',
header: {
type: 'box',
layout: 'vertical',
contents: [
{
type: 'text',
text: 'Header text',
},
],
},
hero: {
type: 'image',
url: 'https://example.com/flex/images/image.jpg',
},
body: {
type: 'box',
layout: 'vertical',
contents: [
{
type: 'text',
text: 'Body text',
},
],
},
footer: {
type: 'box',
layout: 'vertical',
contents: [
{
type: 'text',
text: 'Footer text',
},
],
},
styles: {
comment: 'See the example of a bubble style object',
},
});
Push API - Official Docs
Sends messages to the user, group, or room at any time.
push(messages)
Sends messages to the receiver using ID.
Param | Type | Description |
---|---|---|
messages | Object[] | Array of objects which contains the contents of the message to be sent. |
Example:
context.push([
{
type: 'text',
text: 'Hello!',
},
]);
pushText(text)
- Official Docs
Alias: sendText
.
Sends text message to the receiver using ID.
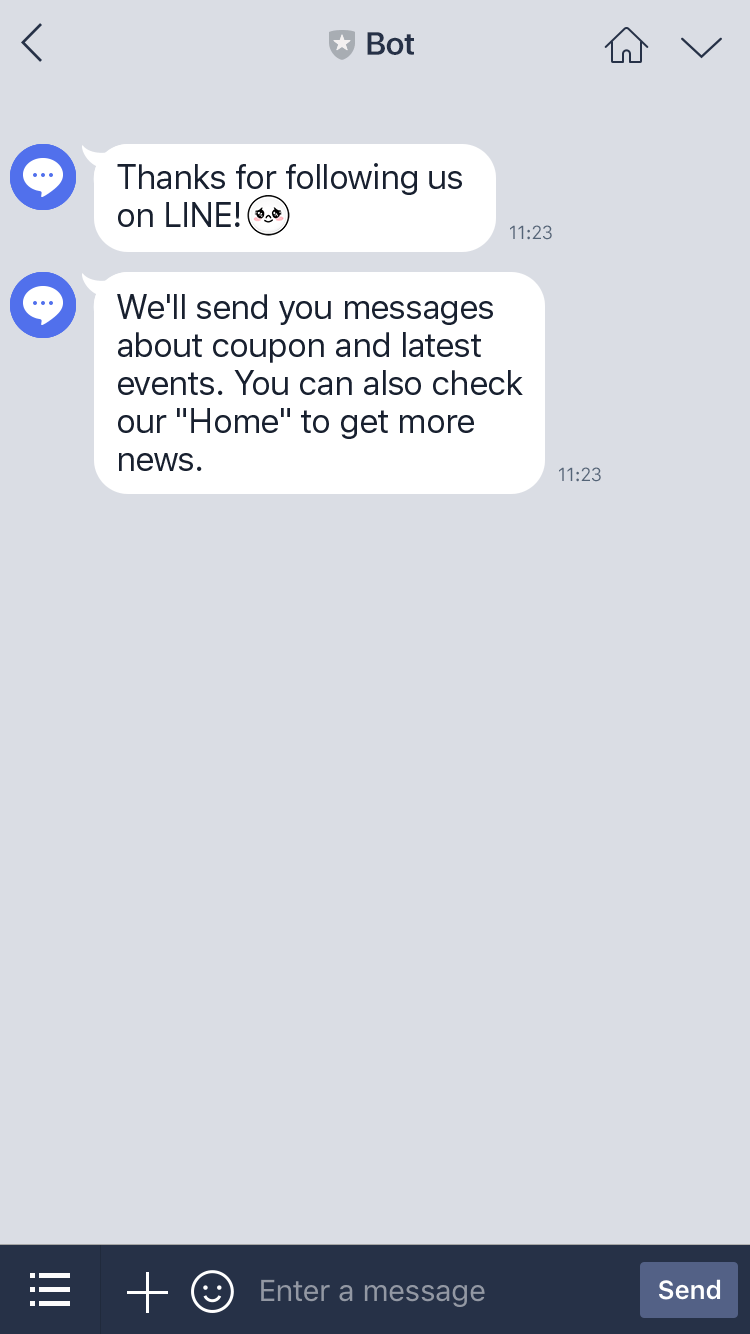
You can include LINE original emoji in text messages using character codes. For a list of LINE emoji that can be sent in LINE chats, see the emoji list.
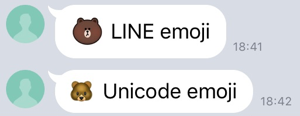
Param | Type | Description |
---|---|---|
text | String | Text of the message to be sent. |
Example:
context.pushText('Hello!');
pushImage(imageUrl, previewImageUrl)
- Official Docs
Alias: sendImage
.
Sends image message to the receiver using ID.
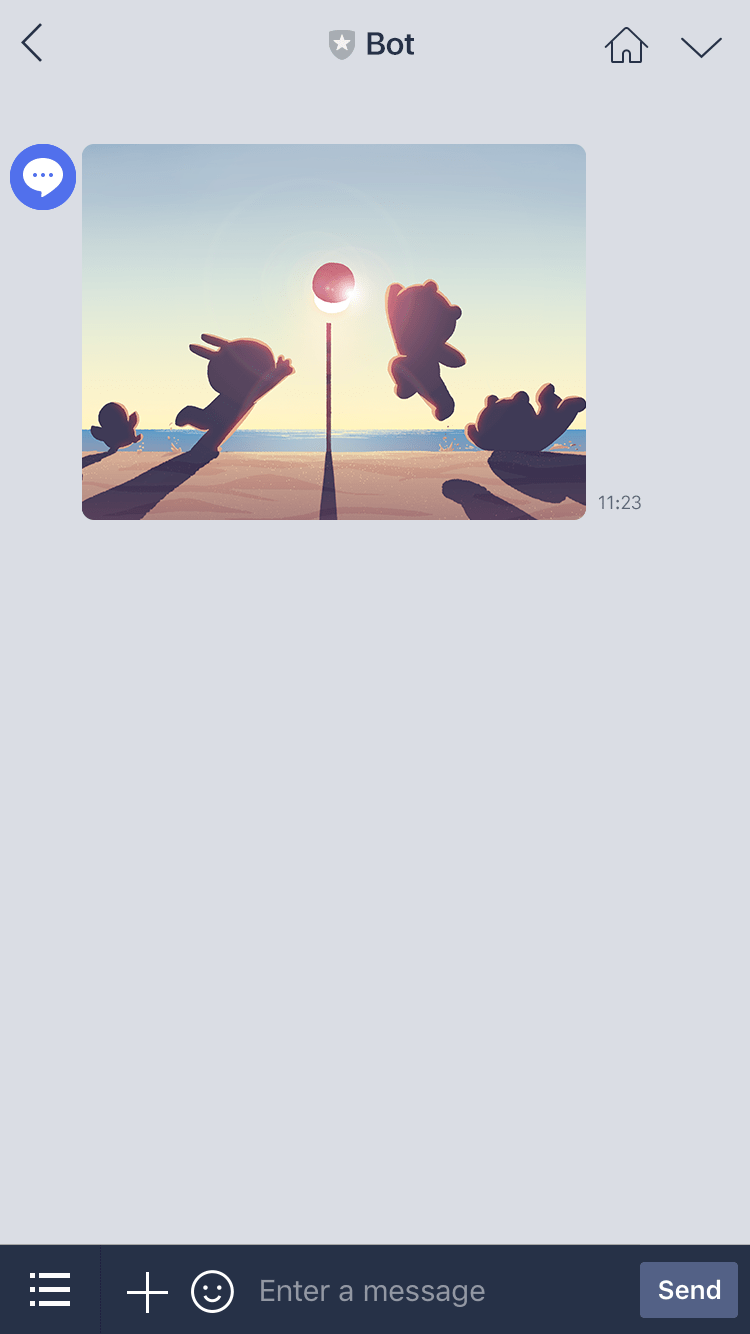
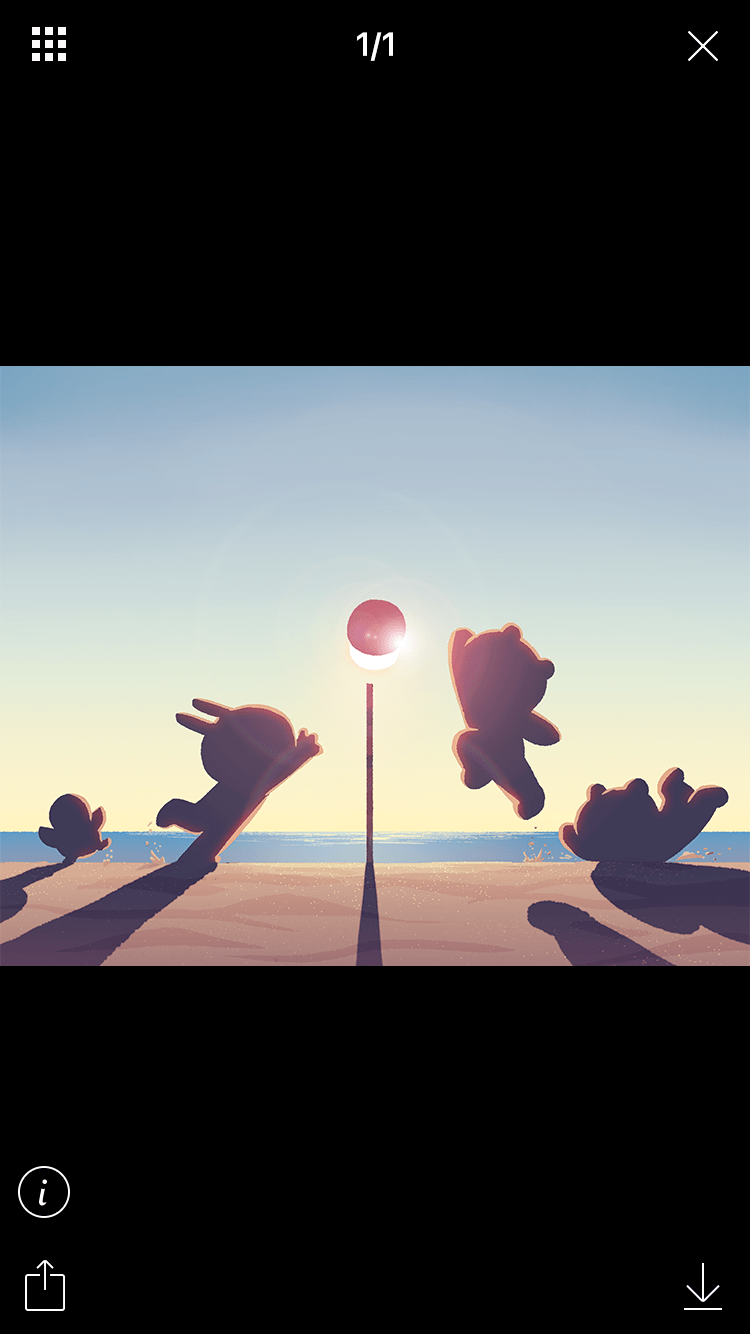
Param | Type | Description |
---|---|---|
imageUrl | String | Image URL. |
previewImageUrl | String | Preview image URL. |
Example:
context.pushImage({
originalContentUrl: 'https://example.com/original.jpg',
previewImageUrl: 'https://example.com/preview.jpg',
});
pushVideo(videoUrl, previewImageUrl)
- Official Docs
Alias: sendVideo
.
Sends video message using ID of the receiver.
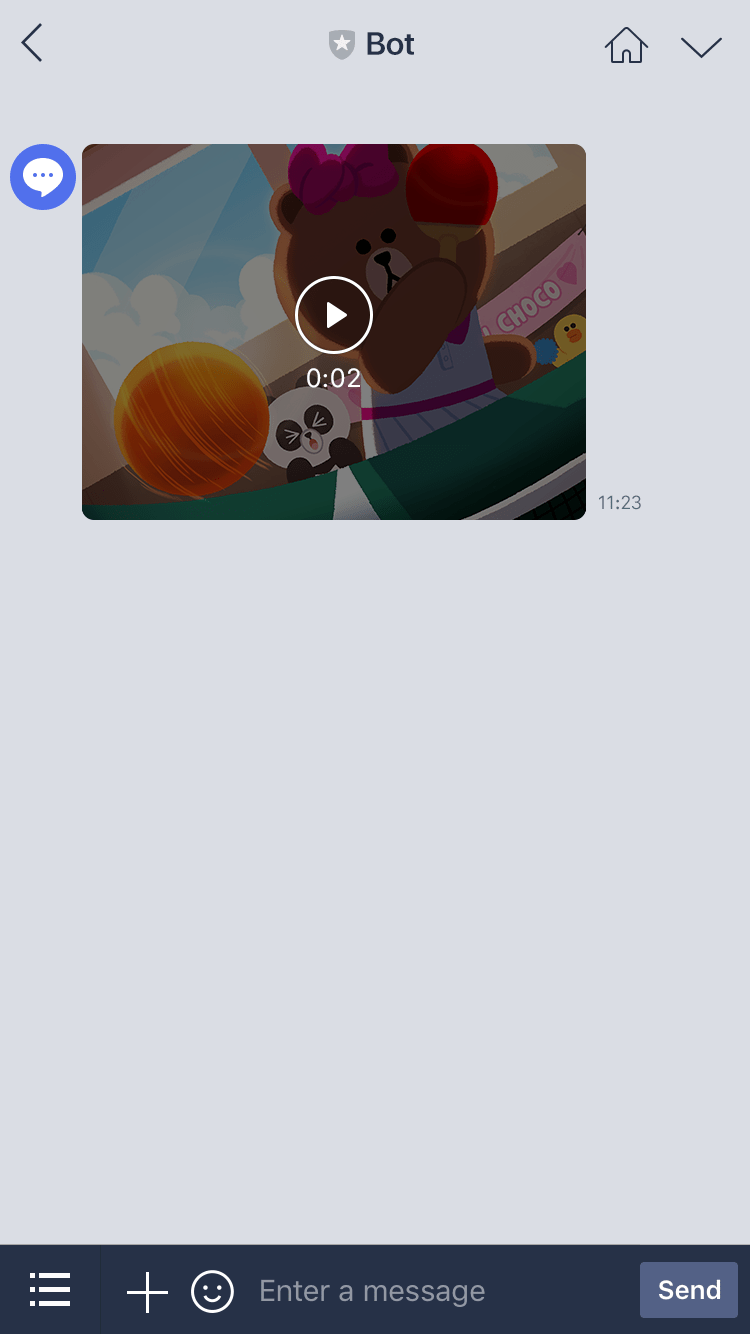
Param | Type | Description |
---|---|---|
videoUrl | String | URL of video file. |
previewImageUrl | String | URL of preview image. |
Example:
context.pushVideo({
originalContentUrl: 'https://example.com/original.mp4',
previewImageUrl: 'https://example.com/preview.jpg',
});
pushAudio(audioUrl, duration)
- Official Docs
Alias: sendAudio
.
Sends audio message to the receiver using ID.
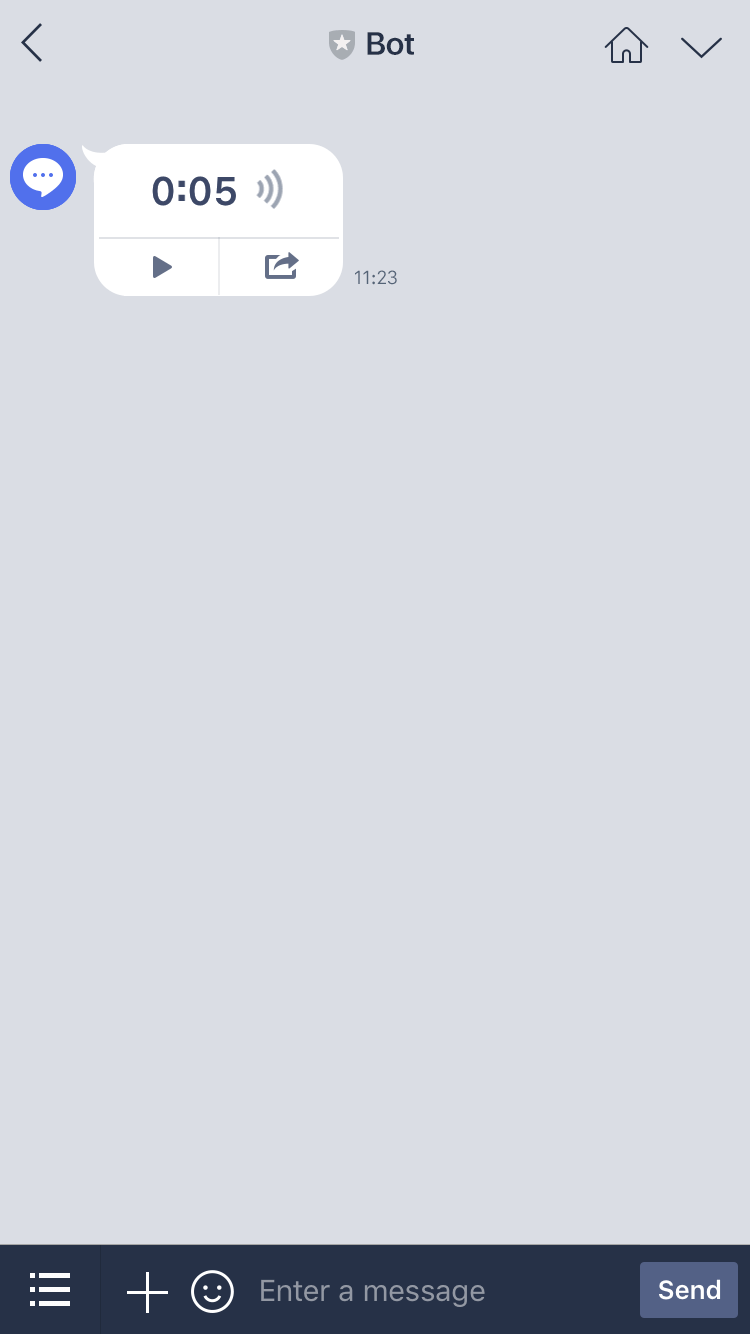
Param | Type | Description |
---|---|---|
audioUrl | String | URL of audio file. |
duration | Number | Length of audio file. |
Example:
context.pushAudio({
originalContentUrl: 'https://example.com/original.m4a',
duration: 240000,
});
pushLocation(location)
- Official Docs
Alias: sendLocation
.
Sends location message to the receiver using ID.
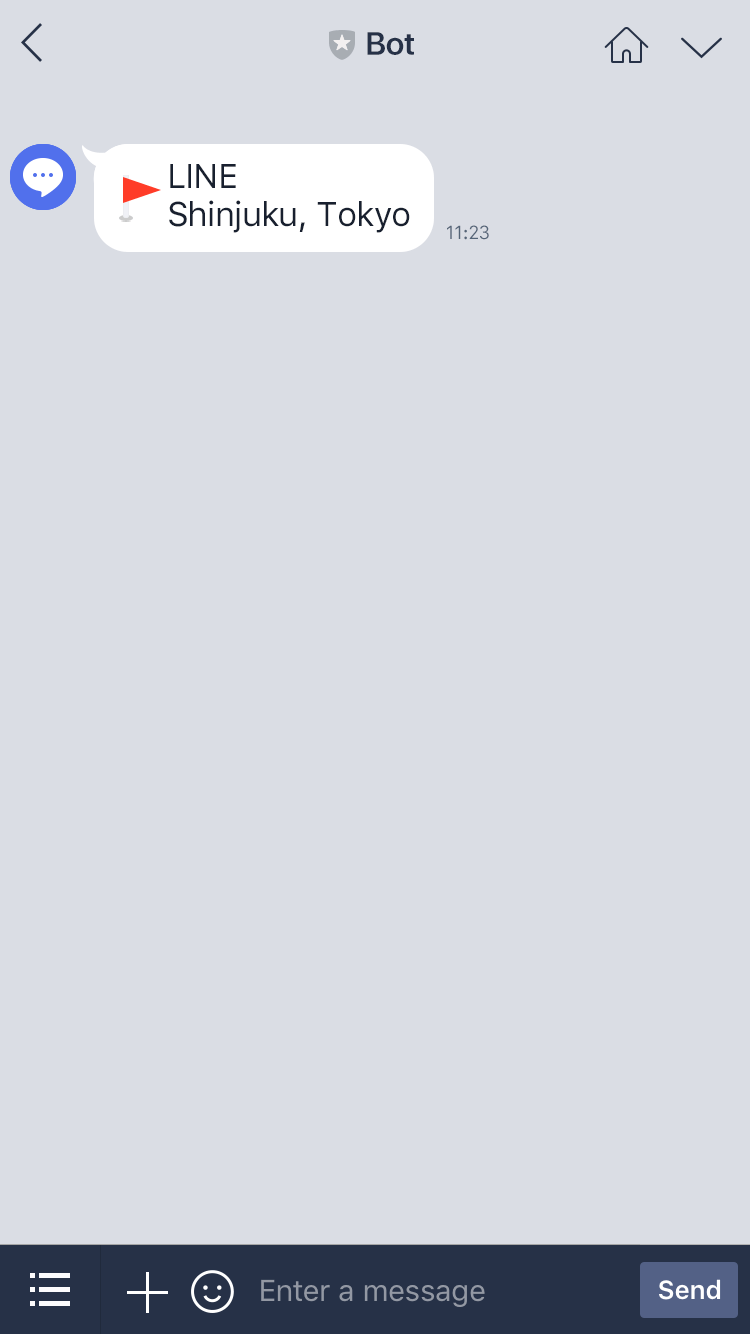
Param | Type | Description |
---|---|---|
location | Object | Object contains location's parameters. |
location.title | String | Title of the location. |
location.address | String | Address of the location. |
location.latitude | Number | Latitude of the location. |
location.longitude | Number | Longitude of the location. |
Example:
context.pushLocation({
title: 'my location',
address: '〒150-0002 東京都渋谷区渋谷2丁目21−1',
latitude: 35.65910807942215,
longitude: 139.70372892916203,
});
pushSticker(packageId, stickerId)
- Official Docs
Alias: sendSticker
.
Sends sticker message to the receiver using ID. For a list of stickers that can be sent with the Messaging API, see the sticker list.
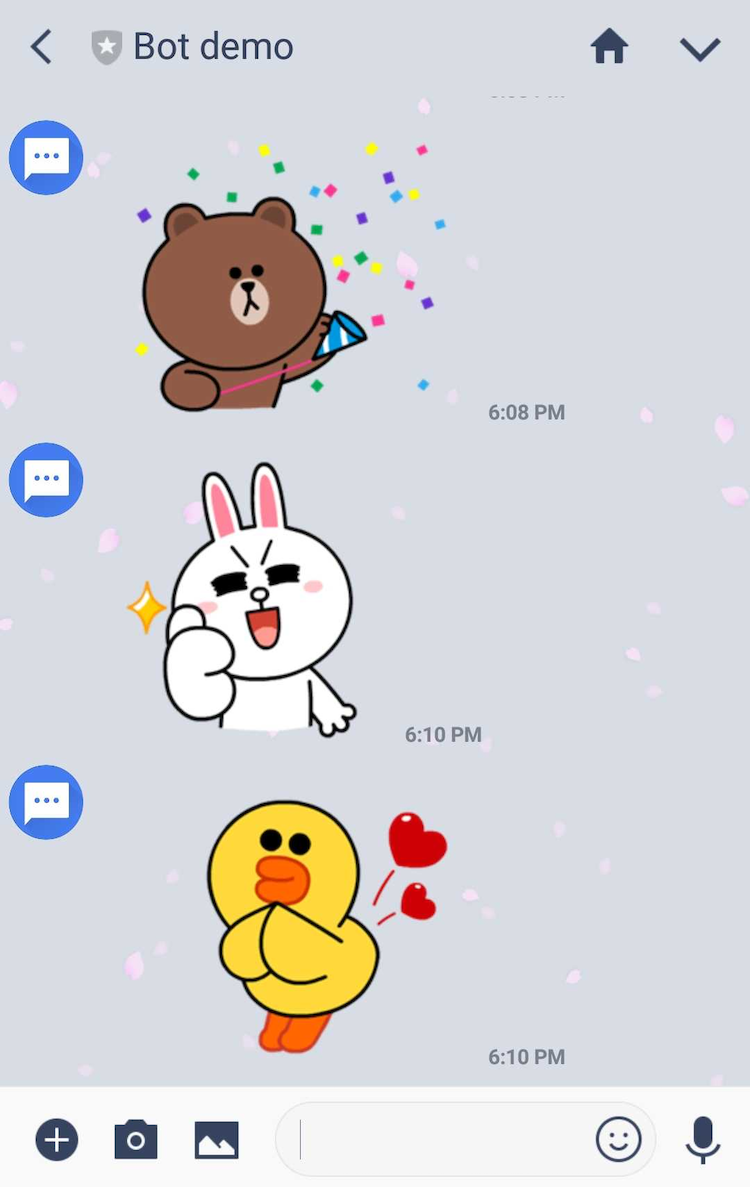
Param | Type | Description |
---|---|---|
packageId | String | Package ID. |
stickerId | String | Sticker ID. |
Example:
context.pushSticker({ packageId: '1', stickerId: '1' });
Push Imagemap Message
pushImagemap(altText, imagemap)
- Official Docs
Alias: sendImagemap
.
Sends imagemap message using ID of the receiver.
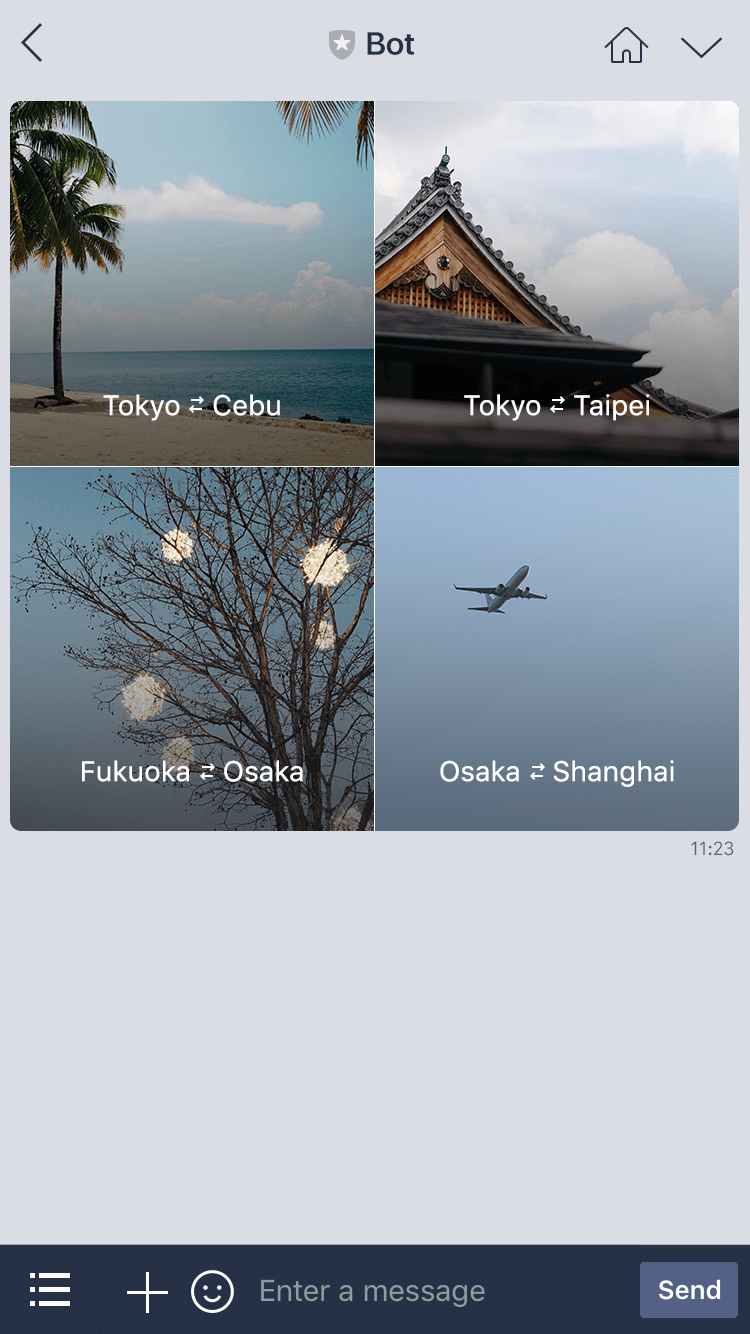
Param | Type | Description |
---|---|---|
altText | String | Alternative text. |
imagemap | Object | Object contains imagemap's parameters. |
imagemap.baseUrl | String | Base URL of image. |
imagemap.baseSize | Object | Base size object. |
imagemap.baseSize.width | Number | Width of base image. |
imagemap.baseSize.height | Number | Height of base image. |
imagemap.actions | Object[] | Action when tapped. |
Example:
context.pushImagemap('this is an imagemap', {
baseUrl: 'https://example.com/bot/images/rm001',
baseSize: {
width: 1040,
height: 1040,
},
actions: [
{
type: 'uri',
linkUri: 'https://example.com/',
area: {
x: 0,
y: 0,
width: 520,
height: 1040,
},
},
{
type: 'message',
text: 'hello',
area: {
x: 520,
y: 0,
width: 520,
height: 1040,
},
},
],
});
Push Template Messages
pushTemplate(altText, template)
- Official Docs
Alias: sendTemplate
.
Sends template message to the receiver using ID.
Param | Type | Description |
---|---|---|
altText | String | Alternative text. |
template | Object | Object with the contents of the template. |
Example:
context.pushTemplate('this is a template', {
type: 'buttons',
thumbnailImageUrl: 'https://example.com/bot/images/image.jpg',
title: 'Menu',
text: 'Please select',
actions: [
{
type: 'postback',
label: 'Buy',
data: 'action=buy&itemid=123',
},
{
type: 'postback',
label: 'Add to cart',
data: 'action=add&itemid=123',
},
{
type: 'uri',
label: 'View detail',
uri: 'http://example.com/page/123',
},
],
});
pushButtonTemplate(altText, buttonTemplate)
- Official Docs
Alias: pushButtonsTemplate
, sendButtonTemplate
, sendButtonsTemplate
.
Sends button template message to the receiver using ID.
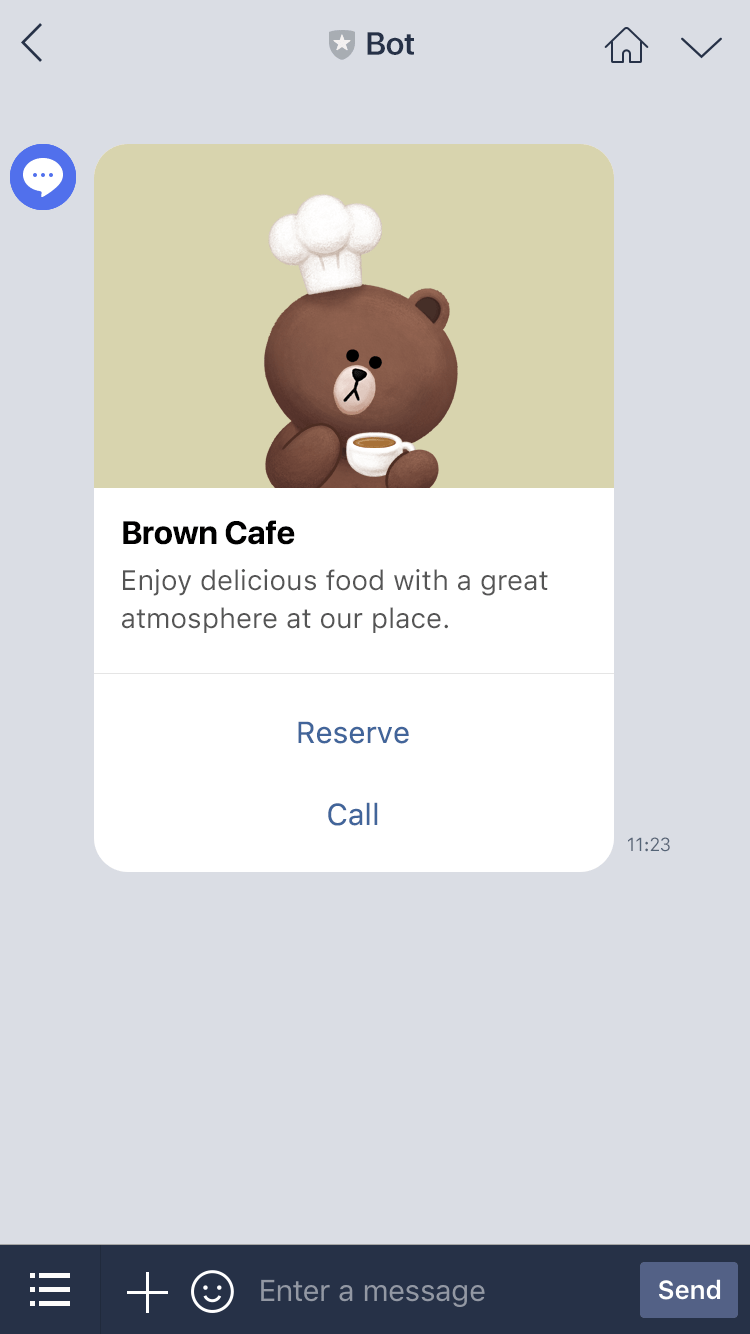
Param | Type | Description |
---|---|---|
altText | String | Alternative text. |
buttonTemplate | Object | Object contains buttonTemplate's parameters. |
buttonTemplate.thumbnailImageUrl | String | Image URL of buttonTemplate. |
buttonTemplate.imageAspectRatio | String | Aspect ratio of the image. Specify one of the following values: rectangle , square |
buttonTemplate.imageSize | String | Size of the image. Specify one of the following values: cover , contain |
buttonTemplate.imageBackgroundColor | String | Background color of image. Specify a RGB color value. The default value is #FFFFFF (white). |
buttonTemplate.title | String | Title of buttonTemplate. |
buttonTemplate.text | String | Message text of buttonTemplate. |
buttonTemplate.defaultAction | Object | Action when image is tapped; set for the entire image, title, and text area. |
buttonTemplate.actions | Object[] | Action when tapped. |
Example:
context.pushButtonTemplate('this is a template', {
thumbnailImageUrl: 'https://example.com/bot/images/image.jpg',
title: 'Menu',
text: 'Please select',
actions: [
{
type: 'postback',
label: 'Buy',
data: 'action=buy&itemid=123',
},
{
type: 'postback',
label: 'Add to cart',
data: 'action=add&itemid=123',
},
{
type: 'uri',
label: 'View detail',
uri: 'http://example.com/page/123',
},
],
});
pushConfirmTemplate(altText, confirmTemplate)
- Official Docs
Alias: sendConfirmTemplate
.
Sends confirm template message using ID of the receiver.
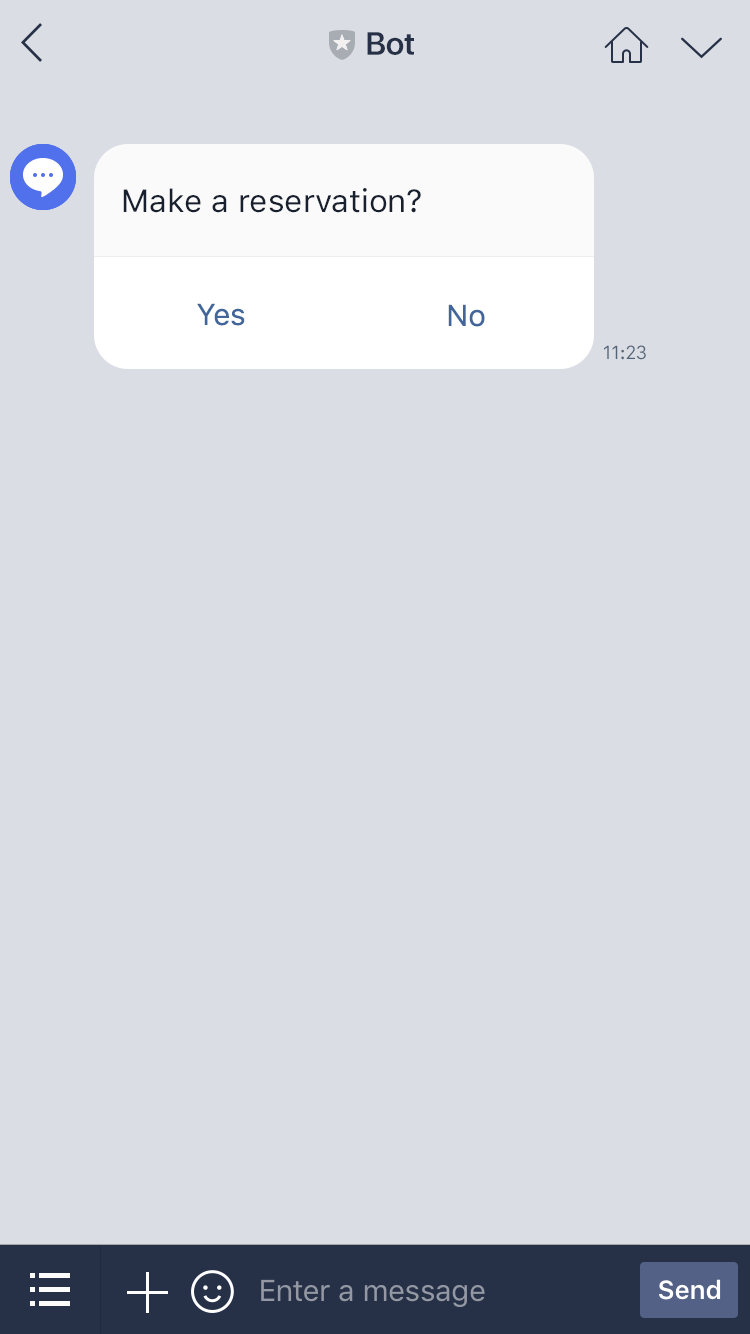
Param | Type | Description |
---|---|---|
altText | String | Alternative text. |
confirmTemplate | Object | Object contains confirmTemplate's parameters. |
confirmTemplate.text | String | Message text of confirmTemplate. |
confirmTemplate.actions | Object[] | Action when tapped. |
Example:
context.pushConfirmTemplate('this is a confirm template', {
text: 'Are you sure?',
actions: [
{
type: 'message',
label: 'Yes',
text: 'yes',
},
{
type: 'message',
label: 'No',
text: 'no',
},
],
});
pushCarouselTemplate(altText, carouselItems, options)
- Official Docs
Alias: sendCarouselTemplate
.
Sends carousel template message to the receiver using ID.
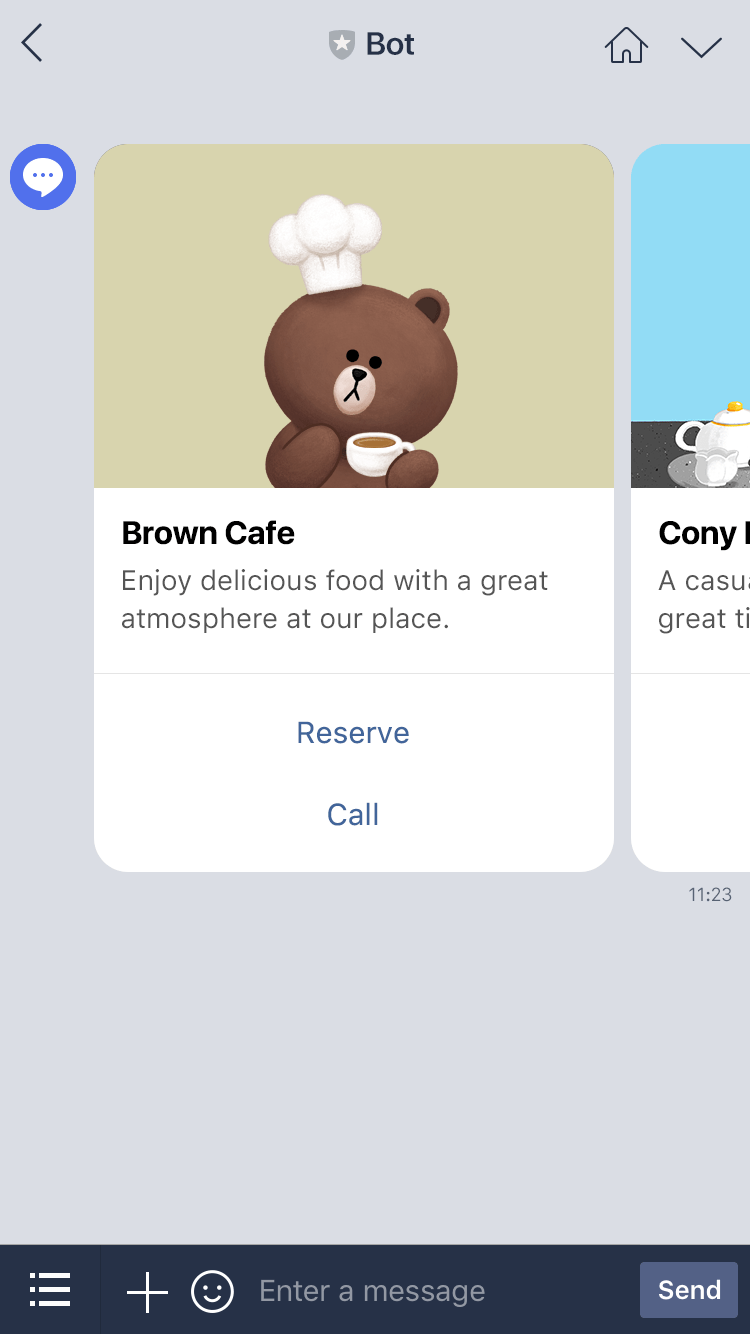
Param | Type | Description |
---|---|---|
altText | String | Alternative text. |
carouselItems | Object[] | Array of columns which contains object for carousel. |
options | Object | Object contains options. |
options.imageAspectRatio | String | Aspect ratio of the image. Specify one of the following values: rectangle , square |
options.imageSize | String | Size of the image. Specify one of the following values: cover , contain |
Example:
context.pushCarouselTemplate('this is a carousel template', [
{
thumbnailImageUrl: 'https://example.com/bot/images/item1.jpg',
title: 'this is menu',
text: 'description',
actions: [
{
type: 'postback',
label: 'Buy',
data: 'action=buy&itemid=111',
},
{
type: 'postback',
label: 'Add to cart',
data: 'action=add&itemid=111',
},
{
type: 'uri',
label: 'View detail',
uri: 'http://example.com/page/111',
},
],
},
{
thumbnailImageUrl: 'https://example.com/bot/images/item2.jpg',
title: 'this is menu',
text: 'description',
actions: [
{
type: 'postback',
label: 'Buy',
data: 'action=buy&itemid=222',
},
{
type: 'postback',
label: 'Add to cart',
data: 'action=add&itemid=222',
},
{
type: 'uri',
label: 'View detail',
uri: 'http://example.com/page/222',
},
],
},
]);
pushImageCarouselTemplate(altText, carouselItems)
- Official Docs
Alias: sendImageCarouselTemplate
.
Sends image carousel template message to the receiver using ID.
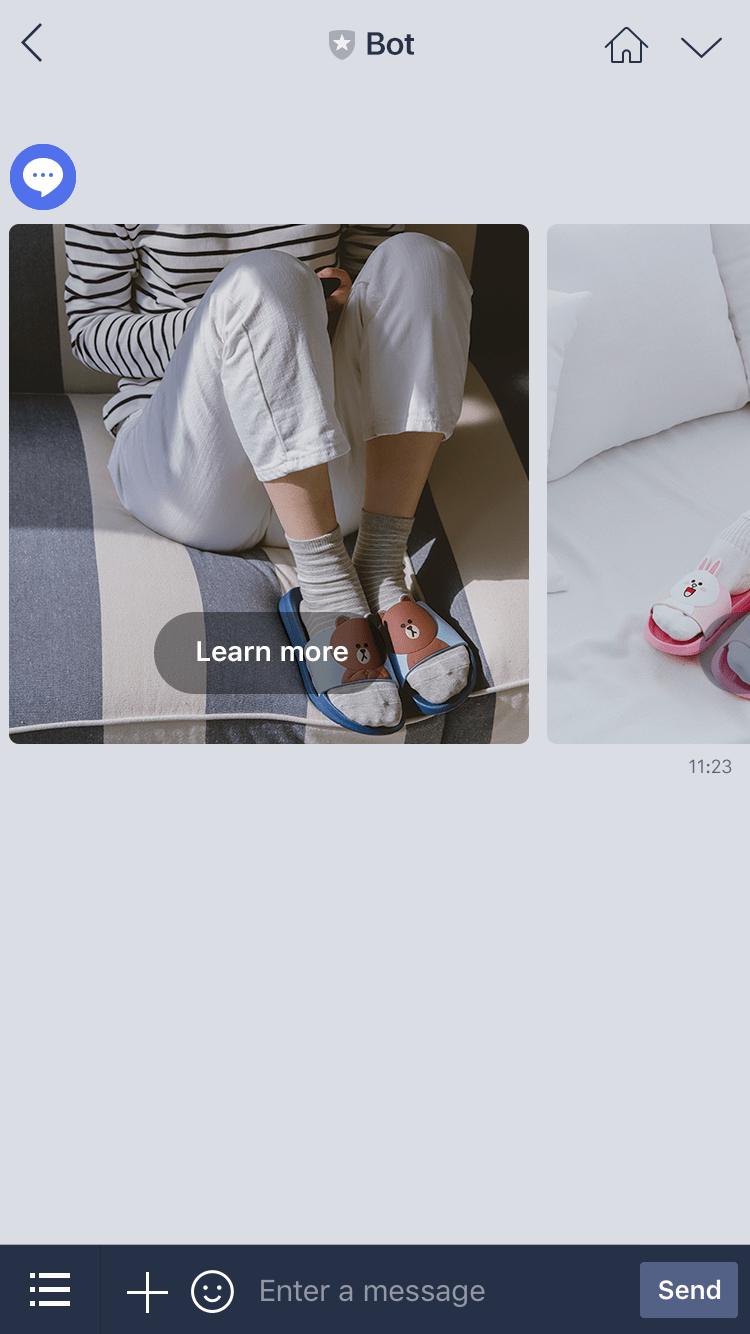
Param | Type | Description |
---|---|---|
altText | String | Alternative text. |
carouselItems | Object[] | Array of columns which contains object for image carousel. |
Example:
context.pushImageCarouselTemplate('this is an image carousel template', [
{
imageUrl: 'https://example.com/bot/images/item1.jpg',
action: {
type: 'postback',
label: 'Buy',
data: 'action=buy&itemid=111',
},
},
{
imageUrl: 'https://example.com/bot/images/item2.jpg',
action: {
type: 'message',
label: 'Yes',
text: 'yes',
},
},
{
imageUrl: 'https://example.com/bot/images/item3.jpg',
action: {
type: 'uri',
label: 'View detail',
uri: 'http://example.com/page/222',
},
},
]);
Push Flex Messages
pushFlex(altText, contents)
- Official Docs
Sends flex message using ID of the receiver.
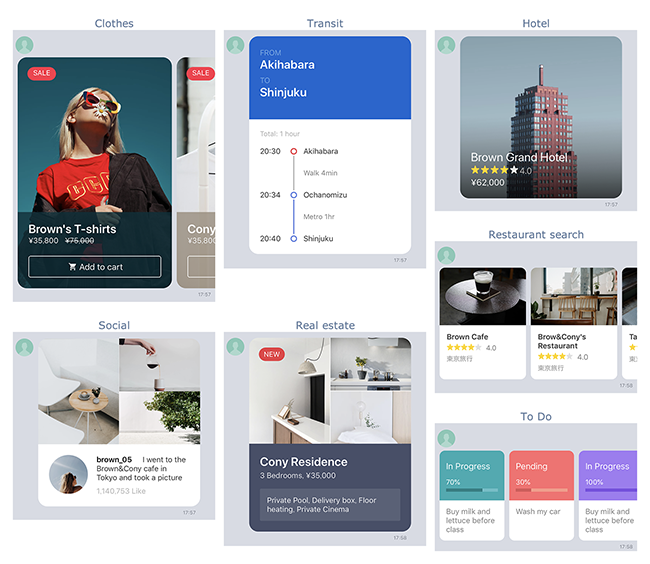
Param | Type | Description |
---|---|---|
altText | String | Alternative text. |
contents | Object | Flex Message container object. |
Example:
context.pushFlex('this is a flex', {
type: 'bubble',
header: {
type: 'box',
layout: 'vertical',
contents: [
{
type: 'text',
text: 'Header text',
},
],
},
hero: {
type: 'image',
url: 'https://example.com/flex/images/image.jpg',
},
body: {
type: 'box',
layout: 'vertical',
contents: [
{
type: 'text',
text: 'Body text',
},
],
},
footer: {
type: 'box',
layout: 'vertical',
contents: [
{
type: 'text',
text: 'Footer text',
},
],
},
styles: {
comment: 'See the example of a bubble style object',
},
});
Quick Replies - Official Docs
Sends message with buttons appear at the bottom of the chat screen.
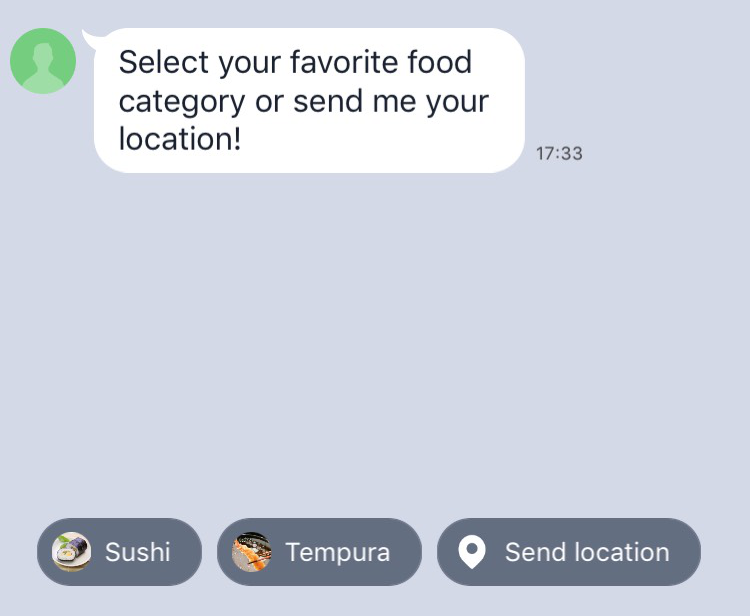
context.replyText(
'Select your favorite food category or send me your location!',
{
quickReply: {
items: [
{
type: 'action',
imageUrl: 'https://example.com/sushi.png',
action: {
type: 'message',
label: 'Sushi',
text: 'Sushi',
},
},
{
type: 'action',
imageUrl: 'https://example.com/tempura.png',
action: {
type: 'message',
label: 'Tempura',
text: 'Tempura',
},
},
{
type: 'action',
action: {
type: 'location',
label: 'Send location',
},
},
],
},
}
);
Profile API - Official Docs
getUserProfile()
Gets user profile information.
Example:
context.getUserProfile().then((profile) => {
console.log(profile);
// {
// displayName: 'LINE taro',
// userId: USER_ID,
// pictureUrl: 'http://obs.line-apps.com/...',
// statusMessage: 'Hello, LINE!',
// }
});
Group/Room Member Profile API - Official Docs
getMemberProfile(userId)
Gets the user profile of a member of the group/room that the bot is in. This includes the user IDs of users who has not added the bot as a friend or has blocked the bot.
Param | Type | Description |
---|---|---|
userId | String | ID of the user. |
Example:
context.getMemberProfile(USER_ID).then((member) => {
console.log(member);
// {
// "displayName":"LINE taro",
// "userId":"Uxxxxxxxxxxxxxx...",
// "pictureUrl":"http://obs.line-apps.com/..."
// }
});
Group/Room Member IDs API - Official Docs
getMemberIds(start)
Gets the ID of the users of the members of the group/room that the bot is in. This includes the user IDs of users who have not added the bot as a friend or has blocked the bot.
This feature is only available for LINE@ Approved accounts or official accounts.
Param | Type | Description |
---|---|---|
start | String | continuationToken . |
Example:
context.getMemberIds(CURSOR).then((res) => {
console.log(res);
// {
// memberIds: [
// 'Uxxxxxxxxxxxxxx...',
// 'Uxxxxxxxxxxxxxx...',
// 'Uxxxxxxxxxxxxxx...'
// ],
// next: 'jxEWCEEP...'
// }
});
getAllMemberIds()
Recursively gets the ID of the users of the members of the group/room that the bot is in using cursors.
This feature is only available for LINE@ Approved accounts or official accounts.
Example:
context.getAllMemberIds().then((ids) => {
console.log(ids);
// [
// 'Uxxxxxxxxxxxxxx..1',
// 'Uxxxxxxxxxxxxxx..2',
// 'Uxxxxxxxxxxxxxx..3',
// 'Uxxxxxxxxxxxxxx..4',
// 'Uxxxxxxxxxxxxxx..5',
// 'Uxxxxxxxxxxxxxx..6',
// ]
});
Leave API - Official Docs
leave()
Leave from the group or room.
Example:
context.leave();
Rich Menu API - Official Docs
getLinkedRichMenu()
- Official Docs
Gets the ID of the rich menu linked to the user.
Example:
context.getLinkedRichMenu().then((richMenu) => {
console.log(richMenu);
// {
// richMenuId: "{richMenuId}"
// }
});
linkRichMenu(richMenuId)
- Official Docs
Links a rich menu to the user.
Param | Type | Description |
---|---|---|
richMenuId | String | ID of an uploaded rich menu. |
Example:
context.linkRichMenu(RICH_MENU_ID);
unlinkRichMenu()
- Official Docs
Unlinks a rich menu from the user.
Example:
context.unlinkRichMenu();
Account Link API - Official Docs
issueLinkToken()
- Official Docs
Issues a link token used for the account link feature.
Example:
context.issueLinkToken().then((result) => {
console.log(result);
// {
// linkToken: 'NMZTNuVrPTqlr2IF8Bnymkb7rXfYv5EY',
// }
});